Selecting single select items in Maximo is piece of cake but configuring multiple select requires some customization.
In this tutorial I will guide in implementing Multiple select in Maximo.
Requirement
I had a requirement to enable Multi-select in a lookup. The user should be able to select Multiple options from the list. All the selected option should be entered in a list as a comma separated list in a single text box.
Implementation
- Add values in Libraries For our this custom multi select menu we need to add some code in Library and Lookup. Go to Application designer click on Select Action drop down. There select export System XML. This will open the following dialog. In the dialog click the arrow against lookups and library. This will download
lookups.xml
andlibrary.xml

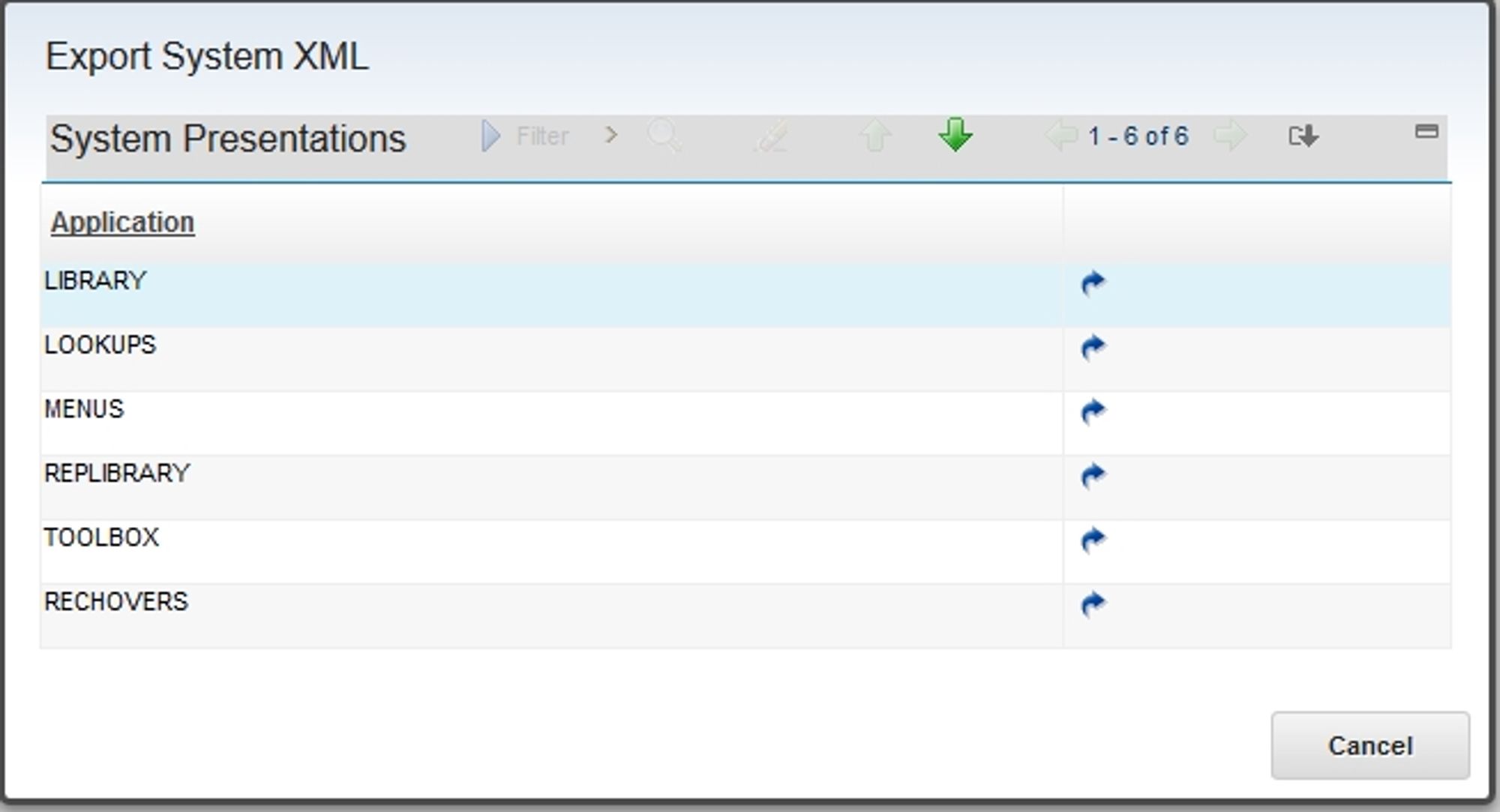
a. Lookup.xml
You can use any lookup any lookup but it should have
selectmode="multiple"
. I created a new lookup. For this open lookups.xml
insert in the bottom of the file the following lines
<table id="multicustomlookup" inputmode="readonly"
b. Library.xml
Open the downloaded
library.xml
In this file search for this code snippet
<dialog beanclass="psdi.webclient.system.beans.LookupBean" icon="img_lookup.gif" id="query_lookup" label="Select Value">
<lookup id="querylookupcontrol"/>
<buttongroup id="querylookup_3">
<pushbutton default="true" id="querylookup_3_1" label="OK" mxevent="dialogok"/>
<pushbutton id="querylookup_3_2" label="Cancel" mxevent="dialogcancel"/>
</buttongroup>
</dialog>
Replace it with the following code. The only change is that change it with your custom bean class which we will write in next section
beanclass="yourpackage.MLMultiSelect"
<dialog
Save the file and import both files (
lookups.xml
and library.xml
) back in to Maximo by using the import application definition in Maximo.- Application Designer
In application designer open the application you need to add multi select lookup into. Select the attribute you want to edit. Right click – Properties. In the lookup field insert
multicustomlookup
. Save the application.
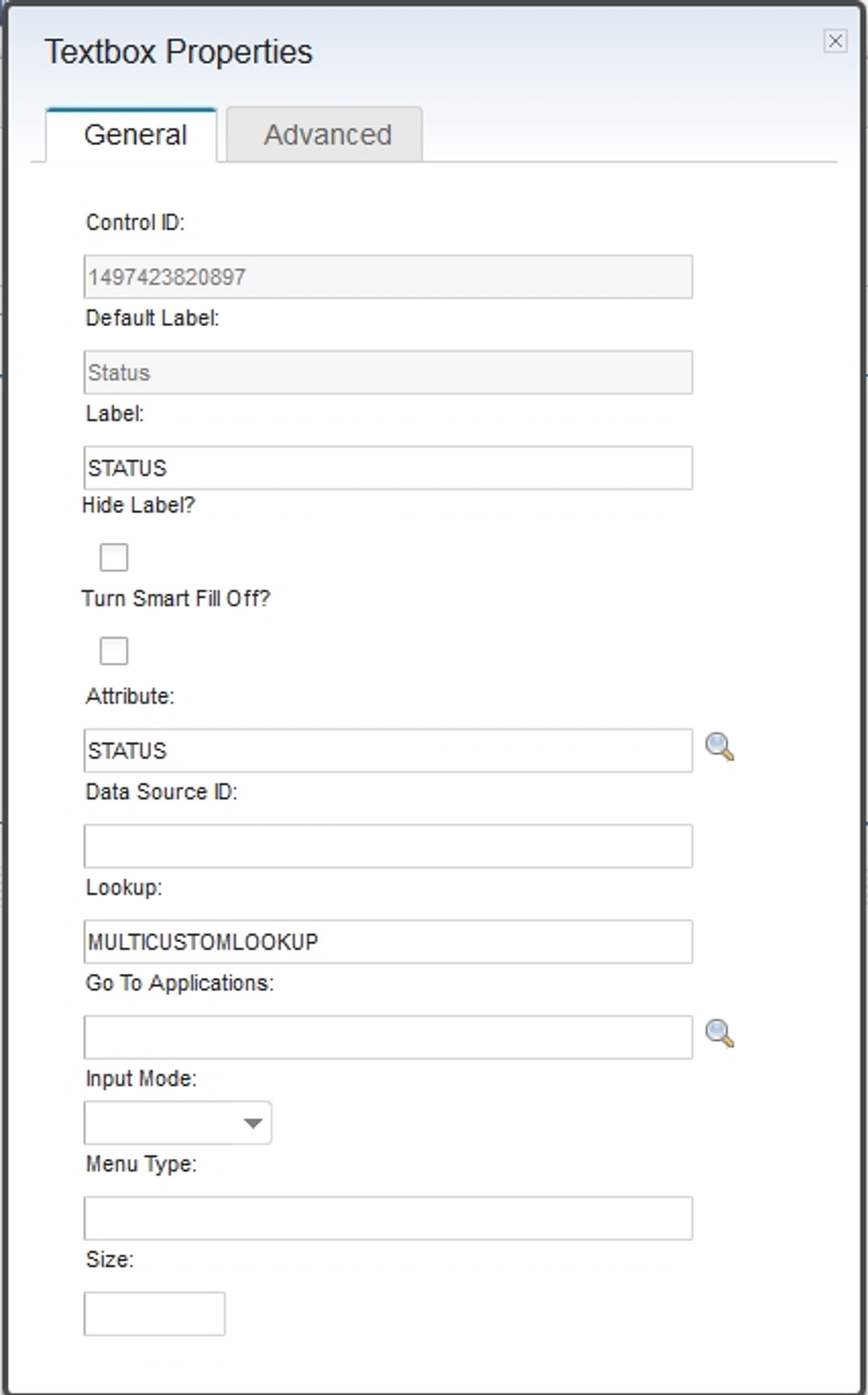
- Java Code Create a new class file. Past the the following code in it.
/**
* Created by Rana Ahmed on 4/25/2016.
* For Multi Selection in Domains
*/
import psdi.mbo.MboConstants;
import psdi.mbo.MboRemote;
import psdi.util.MXException;
import psdi.webclient.system.beans.LookupBean;
import psdi.webclient.system.controller.Utility;
import java.rmi.RemoteException;
import java.util.Iterator;
import java.util.Vector;
public class MLMultiSelectTest extends LookupBean {
private static String comma = ", ";
public void initialize() throws MXException, RemoteException {
/* This is called to select the values when the dialog is initialized. It will loop through the comma seperated values and select them in the dialog.*/
super.initialize();
String fieldToSet = getParent().getReturnAttribute();
MboRemote mbo = this.app.getDataBean().getMbo();
String value = mbo.getString(fieldToSet);
String values[] = value.trim().split("\\s*,\\s*");
for (int i = 0; i < values.length; i++) {
for (int j = 0; j < getMboSet().count(); j++) {
if (values[i].trim().equalsIgnoreCase(getMboSet().getMbo(j).getString("value").trim())) {
getMboSet().select(j);
}
}
}
}
public int execute() throws MXException, RemoteException {
/*This selects the iterates the values and inserts them as a comma seperated list */
boolean onListTab = this.app.onListTab();
if (onListTab)
return super.execute();
String commaString = new String();
String fieldToSet = getParent().getReturnAttribute();
Vector<?> selectedRecords = getMboSet().getSelection();
Iterator<?> selectionIterator = selectedRecords.iterator();
while (selectionIterator.hasNext()) {
MboRemote currentSelectedValue = (MboRemote) selectionIterator.next();
if (commaString.length() == 0)
commaString = currentSelectedValue.getString("VALUE");
else
commaString += comma + currentSelectedValue.getString("VALUE");
}
//custom for Specific Fields
//Use it if you want to process some custom rules.e.g. If a user selects a specific value, he cannot select any other value with it.
//In my requirement if the user selects "Not Applicable" then he should not select any other value with it.
if (fieldToSet.equalsIgnoreCase("MYCUSTOMFIELD") && commaString.contains("Not Applicable")) {
if (!commaString.equalsIgnoreCase("Not Applicable")) {
String parms[] = new String[1];
parms[0] = "ABC";
Utility.showMessageBox(sessionContext.getCurrentEvent(), "myapp", "notapplicable", parms);
return 0;
}
}
//End Custom Specific Rules
MboRemote mbo = this.app.getDataBean().getMbo();
mbo.setValue(fieldToSet, commaString, MboConstants.NOVALIDATION);
app.getAppBean().refreshTable();
return 1;
}
}
4. Deploy
Deploy the code and restart Maximo application server
5. Check
Open you application and try selecting some multi select values. It should now paste them as comma separated list.

Click again on lookup icon and it should display those comma separated values as selected values.
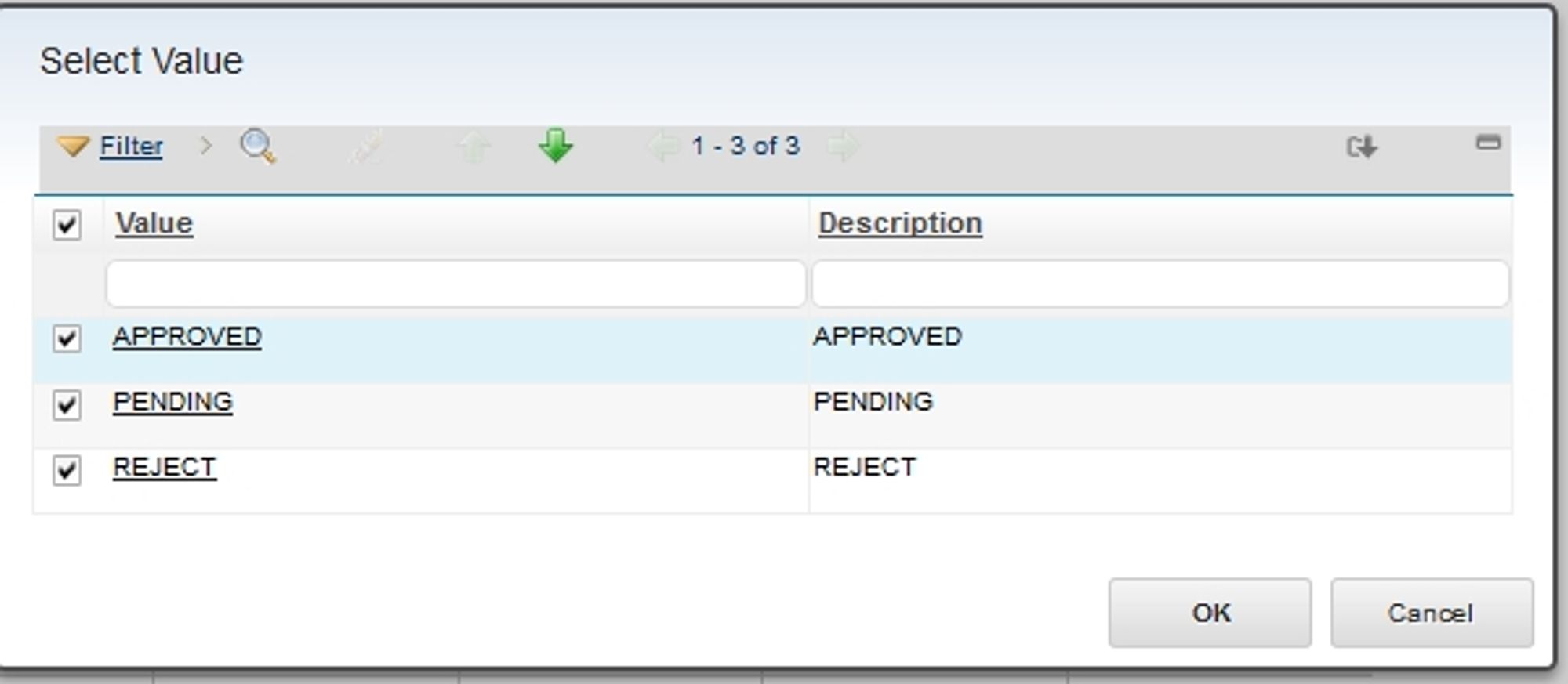
Thanks to this blog for much help